Exploring data#
When working with a dataset, the first step is usually to understand what data and metadata it contains. In this chapter we explore how Scipp supports this.
This tutorial contains exercises, but solutions are included directly. We encourage you to download this notebook and run through it step by step before looking at the solutions. We recommend to use a recent version of JupyterLab: The solutions are included as hidden cells and shown only on demand.
First, in addition to importing scipp
, we import scippneutron
for neutron-science routines.
[1]:
import scipp as sc
import scippneutron as scn
import scippneutron.data
import plopp as pp
We start by loading some data, in this case measured with a prototype of the LoKI detectors at the LARMOR beamline:
[2]:
data = scn.data.tutorial_dense_data()
In practice, we would use ScippNeXus or scippneutron.load_with_mantid to load the data from a NeXus file, but the tutorial data comes bundled with ScippNeutron to make it easily available. See Tutorial and Test Data for a way to customize where the data is stored.
Note that the exercises in the following are fictional and do not represent the actual SANS data reduction workflow.
Step 1: Use the HTML representation to see what the loaded data contains#
The HTML representation is what Jupyter displays for a scipp object.
Take some time to explore this view and try to understand all the information (dimensions, dtypes, units, …).
Note that sections can be expanded, and values can shown by clicking the icons to the right.
[3]:
data
[3]:
- detectorscippDataArray(spectrum: 114688, tof: 200)float64counts0.0, 0.0, ..., 0.0, 0.0
- scippDataGroup(tof: 200)
- monitor1scippDataArray(tof: 200)float64counts583.995, 112.997, ..., 75.196, 4036.448
- monitor2scippDataArray(tof: 200)float64counts45.997, 42.998, ..., 46.849, 36.547
- monitor3scippDataArray(tof: 200)float64counts0.0, 0.0, ..., 0.0, 0.0
- monitor4scippDataArray(tof: 200)float64counts359.964, 502.005, ..., 50.146, 71.448
- monitor5scippDataArray(tof: 200)float64counts0.0, 0.0, ..., 0.0, 0.0
- proton_charge_by_periodscippVariable()float64𝟙0.3437764048576355
- gd_prtn_chrgscippVariable()float64𝟙2.750211238861084
- DCMagField1scippDataArray(time: 24)float64𝟙-0.002, -0.002, ..., -0.002, -0.002
- DCMagField2scippDataArray(time: 24)float64𝟙0.0, 0.0, ..., 0.0, 0.0
- DCMagField3scippDataArray(time: 24)float64𝟙-1.085, -1.085, ..., -1.085, -1.085
- DCMagField4scippDataArray(time: 24)float64𝟙-1.752, -1.752, ..., -1.752, -1.752
- instrument_namestr()LARMOR
- run_endstr()2019-12-18T18:36:41
- run_startstr()2019-12-18T17:36:11
- run_headerstr()LAR 49293 Dalgliesh,Raspino,Ho LOKI Detector Test Dumm 16-DEC-2019 17:53:49 ...
- run_statusscippDataArray(time: 2)int32𝟙1, 1
- run_numberstr()49338
[4]:
detector = data['detector']
detector
[4]:
- spectrum: 114688
- tof: 200
- position(spectrum)vector3m[ 0.778 0.13046651 29.85877813], [ 0.77506458 0.13046651 29.85877813], ..., [-5.69651663e-01 -2.28657089e-02 2.99532831e+01], [-5.72000000e-01 -2.28657089e-02 2.99532831e+01]
Values:
array([[ 7.78000000e-01, 1.30466508e-01, 2.98587781e+01], [ 7.75064579e-01, 1.30466508e-01, 2.98587781e+01], [ 7.72129159e-01, 1.30466508e-01, 2.98587781e+01], ..., [-5.67303327e-01, -2.28657089e-02, 2.99532831e+01], [-5.69651663e-01, -2.28657089e-02, 2.99532831e+01], [-5.72000000e-01, -2.28657089e-02, 2.99532831e+01]]) - sample_position()vector3m[ 0. 0. 25.3]
Values:
array([ 0. , 0. , 25.3]) - source_position()vector3m[0. 0. 0.]
Values:
array([0., 0., 0.]) - spectrum(spectrum)int32𝟙11, 12, ..., 114697, 114698
Values:
array([ 11, 12, 13, ..., 114696, 114697, 114698], dtype=int32) - tof(tof [bin-edge])float64µs5.0, 504.975, ..., 9.950e+04, 1.000e+05
Values:
array([5.0000000e+00, 5.0497500e+02, 1.0049500e+03, 1.5049250e+03, 2.0049000e+03, 2.5048750e+03, 3.0048500e+03, 3.5048250e+03, 4.0048000e+03, 4.5047750e+03, 5.0047500e+03, 5.5047250e+03, 6.0047000e+03, 6.5046750e+03, 7.0046500e+03, 7.5046250e+03, 8.0046000e+03, 8.5045750e+03, 9.0045500e+03, 9.5045250e+03, 1.0004500e+04, 1.0504475e+04, 1.1004450e+04, 1.1504425e+04, 1.2004400e+04, 1.2504375e+04, 1.3004350e+04, 1.3504325e+04, 1.4004300e+04, 1.4504275e+04, 1.5004250e+04, 1.5504225e+04, 1.6004200e+04, 1.6504175e+04, 1.7004150e+04, 1.7504125e+04, 1.8004100e+04, 1.8504075e+04, 1.9004050e+04, 1.9504025e+04, 2.0004000e+04, 2.0503975e+04, 2.1003950e+04, 2.1503925e+04, 2.2003900e+04, 2.2503875e+04, 2.3003850e+04, 2.3503825e+04, 2.4003800e+04, 2.4503775e+04, 2.5003750e+04, 2.5503725e+04, 2.6003700e+04, 2.6503675e+04, 2.7003650e+04, 2.7503625e+04, 2.8003600e+04, 2.8503575e+04, 2.9003550e+04, 2.9503525e+04, 3.0003500e+04, 3.0503475e+04, 3.1003450e+04, 3.1503425e+04, 3.2003400e+04, 3.2503375e+04, 3.3003350e+04, 3.3503325e+04, 3.4003300e+04, 3.4503275e+04, 3.5003250e+04, 3.5503225e+04, 3.6003200e+04, 3.6503175e+04, 3.7003150e+04, 3.7503125e+04, 3.8003100e+04, 3.8503075e+04, 3.9003050e+04, 3.9503025e+04, 4.0003000e+04, 4.0502975e+04, 4.1002950e+04, 4.1502925e+04, 4.2002900e+04, 4.2502875e+04, 4.3002850e+04, 4.3502825e+04, 4.4002800e+04, 4.4502775e+04, 4.5002750e+04, 4.5502725e+04, 4.6002700e+04, 4.6502675e+04, 4.7002650e+04, 4.7502625e+04, 4.8002600e+04, 4.8502575e+04, 4.9002550e+04, 4.9502525e+04, 5.0002500e+04, 5.0502475e+04, 5.1002450e+04, 5.1502425e+04, 5.2002400e+04, 5.2502375e+04, 5.3002350e+04, 5.3502325e+04, 5.4002300e+04, 5.4502275e+04, 5.5002250e+04, 5.5502225e+04, 5.6002200e+04, 5.6502175e+04, 5.7002150e+04, 5.7502125e+04, 5.8002100e+04, 5.8502075e+04, 5.9002050e+04, 5.9502025e+04, 6.0002000e+04, 6.0501975e+04, 6.1001950e+04, 6.1501925e+04, 6.2001900e+04, 6.2501875e+04, 6.3001850e+04, 6.3501825e+04, 6.4001800e+04, 6.4501775e+04, 6.5001750e+04, 6.5501725e+04, 6.6001700e+04, 6.6501675e+04, 6.7001650e+04, 6.7501625e+04, 6.8001600e+04, 6.8501575e+04, 6.9001550e+04, 6.9501525e+04, 7.0001500e+04, 7.0501475e+04, 7.1001450e+04, 7.1501425e+04, 7.2001400e+04, 7.2501375e+04, 7.3001350e+04, 7.3501325e+04, 7.4001300e+04, 7.4501275e+04, 7.5001250e+04, 7.5501225e+04, 7.6001200e+04, 7.6501175e+04, 7.7001150e+04, 7.7501125e+04, 7.8001100e+04, 7.8501075e+04, 7.9001050e+04, 7.9501025e+04, 8.0001000e+04, 8.0500975e+04, 8.1000950e+04, 8.1500925e+04, 8.2000900e+04, 8.2500875e+04, 8.3000850e+04, 8.3500825e+04, 8.4000800e+04, 8.4500775e+04, 8.5000750e+04, 8.5500725e+04, 8.6000700e+04, 8.6500675e+04, 8.7000650e+04, 8.7500625e+04, 8.8000600e+04, 8.8500575e+04, 8.9000550e+04, 8.9500525e+04, 9.0000500e+04, 9.0500475e+04, 9.1000450e+04, 9.1500425e+04, 9.2000400e+04, 9.2500375e+04, 9.3000350e+04, 9.3500325e+04, 9.4000300e+04, 9.4500275e+04, 9.5000250e+04, 9.5500225e+04, 9.6000200e+04, 9.6500175e+04, 9.7000150e+04, 9.7500125e+04, 9.8000100e+04, 9.8500075e+04, 9.9000050e+04, 9.9500025e+04, 1.0000000e+05])
- (spectrum, tof)float64counts0.0, 0.0, ..., 0.0, 0.0σ = 0.0, 0.0, ..., 0.0, 0.0
Values:
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
Variances (σ²):
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
Whenever you get stuck with one of the exercises below we recommend consulting the HTML representations of the objects you are working with.
Step 2: Plot the data#
Scipp objects (variables, data arrays, datasets, or data groups) can be plotted using the plot()
method. Alternatively sc.plot(obj)
can be used, e.g., when obj
is a Python dict
of scipp data arrays. Since this is neutron-scattering data, we can also use the “instrument view”, provided by scn.instrument_view(obj)
(assuming scippneutron
was imported as scn
).
Exercise#
Plot the loaded data and familiarize yourself with the controls.
Create the instrument view and familiarize yourself with the controls.
Solution#
[5]:
detector.sum('spectrum').plot()
[5]:
[6]:
scn.instrument_view(detector.sum('tof'), norm='log')
[6]:
Step 3: Exploring metadata#
Above we saw that the input data group contains a number of metadata items in addition to the the main ‘detector’. Some items are simple strings while others are data arrays or variables. These have various meanings and we now want to explore them.
Exercise#
Find some data array items of
data
and plot them. Also trysc.table(item)
to show a table representation (whereitem
is an item of your choice).Find and plot a monitor.
Try to normalize
detector
to monitor 1. Why does this fail?Plot all the monitors on the same plot. Note that
sc.plot()
can be used with a data group.Convert all the monitors from
'tof'
to'wavelength'
using, e.g.,wavelength_graph_monitor = { **scn.conversion.graph.beamline.beamline(scatter=False), **scn.conversion.graph.tof.elastic_wavelength('tof'), } mon1_wav = mon1.transform_coords('wavelength', graph=wavelength_graph_monitor)
Inspect the HTML view and note how the “unit conversion” changed the dimensions and units.
Re-plot all the monitors on the same plot, now in
'wavelength'
.
Solution#
[7]:
sc.table(data['DCMagField2'])
[7]:
Coordinates | Data |
---|---|
time [ns] | [𝟙] |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:14.000000000 | 0.000 |
2019-12-16T17:53:51.000000000 | 0.000 |
2019-12-16T17:53:51.000000000 | 0.000 |
... | ... |
2019-12-16T17:53:51.000000000 | 0.000 |
2019-12-16T17:53:51.000000000 | 0.000 |
2019-12-18T11:11:16.000000000 | 0.000 |
2019-12-18T18:36:41.000000000 | 0.000 |
2019-12-19T01:11:41.000000000 | 0.000 |
2019-12-19T06:22:07.000000000 | 0.000 |
2019-12-19T12:14:46.000000000 | 0.000 |
2019-12-19T17:18:58.000000000 | 0.000 |
2019-12-19T22:22:32.000000000 | 0.000 |
2019-12-20T03:26:14.000000000 | 0.000 |
[8]:
detector / data['monitors']['monitor1']
---------------------------------------------------------------------------
DatasetError Traceback (most recent call last)
Cell In[8], line 1
----> 1 detector / data['monitors']['monitor1']
DatasetError: Mismatch in coordinate 'position' in operation 'divide':
(spectrum: 114688) vector3 [m] [(0.778, 0.130467, 29.8588), (0.775065, 0.130467, 29.8588), ..., (-0.569652, -0.0228657, 29.9533), (-0.572, -0.0228657, 29.9533)]
vs
() vector3 [m] (0, 0, 9.8195)
A monitor can be converted to wavelength as follows:
[9]:
wavelength_graph_monitor = {
**scn.conversion.graph.beamline.beamline(scatter=False),
**scn.conversion.graph.tof.elastic_wavelength('tof'),
}
[10]:
mon1 = data['monitors']['monitor1']
mon1.transform_coords('wavelength', graph=wavelength_graph_monitor)
[10]:
- wavelength: 200
- Ltotal()float64m9.8195
Values:
array(9.8195) - position()vector3m[0. 0. 9.8195]
Values:
array([0. , 0. , 9.8195]) - sample_position()vector3m[ 0. 0. 25.3]
Values:
array([ 0. , 0. , 25.3]) - source_position()vector3m[0. 0. 0.]
Values:
array([0., 0., 0.]) - spectrum()int32𝟙1
Values:
array(1, dtype=int32) - tof(wavelength [bin-edge])float64µs5.0, 504.975, ..., 9.950e+04, 1.000e+05
Values:
array([5.0000000e+00, 5.0497500e+02, 1.0049500e+03, 1.5049250e+03, 2.0049000e+03, 2.5048750e+03, 3.0048500e+03, 3.5048250e+03, 4.0048000e+03, 4.5047750e+03, 5.0047500e+03, 5.5047250e+03, 6.0047000e+03, 6.5046750e+03, 7.0046500e+03, 7.5046250e+03, 8.0046000e+03, 8.5045750e+03, 9.0045500e+03, 9.5045250e+03, 1.0004500e+04, 1.0504475e+04, 1.1004450e+04, 1.1504425e+04, 1.2004400e+04, 1.2504375e+04, 1.3004350e+04, 1.3504325e+04, 1.4004300e+04, 1.4504275e+04, 1.5004250e+04, 1.5504225e+04, 1.6004200e+04, 1.6504175e+04, 1.7004150e+04, 1.7504125e+04, 1.8004100e+04, 1.8504075e+04, 1.9004050e+04, 1.9504025e+04, 2.0004000e+04, 2.0503975e+04, 2.1003950e+04, 2.1503925e+04, 2.2003900e+04, 2.2503875e+04, 2.3003850e+04, 2.3503825e+04, 2.4003800e+04, 2.4503775e+04, 2.5003750e+04, 2.5503725e+04, 2.6003700e+04, 2.6503675e+04, 2.7003650e+04, 2.7503625e+04, 2.8003600e+04, 2.8503575e+04, 2.9003550e+04, 2.9503525e+04, 3.0003500e+04, 3.0503475e+04, 3.1003450e+04, 3.1503425e+04, 3.2003400e+04, 3.2503375e+04, 3.3003350e+04, 3.3503325e+04, 3.4003300e+04, 3.4503275e+04, 3.5003250e+04, 3.5503225e+04, 3.6003200e+04, 3.6503175e+04, 3.7003150e+04, 3.7503125e+04, 3.8003100e+04, 3.8503075e+04, 3.9003050e+04, 3.9503025e+04, 4.0003000e+04, 4.0502975e+04, 4.1002950e+04, 4.1502925e+04, 4.2002900e+04, 4.2502875e+04, 4.3002850e+04, 4.3502825e+04, 4.4002800e+04, 4.4502775e+04, 4.5002750e+04, 4.5502725e+04, 4.6002700e+04, 4.6502675e+04, 4.7002650e+04, 4.7502625e+04, 4.8002600e+04, 4.8502575e+04, 4.9002550e+04, 4.9502525e+04, 5.0002500e+04, 5.0502475e+04, 5.1002450e+04, 5.1502425e+04, 5.2002400e+04, 5.2502375e+04, 5.3002350e+04, 5.3502325e+04, 5.4002300e+04, 5.4502275e+04, 5.5002250e+04, 5.5502225e+04, 5.6002200e+04, 5.6502175e+04, 5.7002150e+04, 5.7502125e+04, 5.8002100e+04, 5.8502075e+04, 5.9002050e+04, 5.9502025e+04, 6.0002000e+04, 6.0501975e+04, 6.1001950e+04, 6.1501925e+04, 6.2001900e+04, 6.2501875e+04, 6.3001850e+04, 6.3501825e+04, 6.4001800e+04, 6.4501775e+04, 6.5001750e+04, 6.5501725e+04, 6.6001700e+04, 6.6501675e+04, 6.7001650e+04, 6.7501625e+04, 6.8001600e+04, 6.8501575e+04, 6.9001550e+04, 6.9501525e+04, 7.0001500e+04, 7.0501475e+04, 7.1001450e+04, 7.1501425e+04, 7.2001400e+04, 7.2501375e+04, 7.3001350e+04, 7.3501325e+04, 7.4001300e+04, 7.4501275e+04, 7.5001250e+04, 7.5501225e+04, 7.6001200e+04, 7.6501175e+04, 7.7001150e+04, 7.7501125e+04, 7.8001100e+04, 7.8501075e+04, 7.9001050e+04, 7.9501025e+04, 8.0001000e+04, 8.0500975e+04, 8.1000950e+04, 8.1500925e+04, 8.2000900e+04, 8.2500875e+04, 8.3000850e+04, 8.3500825e+04, 8.4000800e+04, 8.4500775e+04, 8.5000750e+04, 8.5500725e+04, 8.6000700e+04, 8.6500675e+04, 8.7000650e+04, 8.7500625e+04, 8.8000600e+04, 8.8500575e+04, 8.9000550e+04, 8.9500525e+04, 9.0000500e+04, 9.0500475e+04, 9.1000450e+04, 9.1500425e+04, 9.2000400e+04, 9.2500375e+04, 9.3000350e+04, 9.3500325e+04, 9.4000300e+04, 9.4500275e+04, 9.5000250e+04, 9.5500225e+04, 9.6000200e+04, 9.6500175e+04, 9.7000150e+04, 9.7500125e+04, 9.8000100e+04, 9.8500075e+04, 9.9000050e+04, 9.9500025e+04, 1.0000000e+05]) - wavelength(wavelength [bin-edge])float64Å0.002, 0.203, ..., 40.086, 40.288
Values:
array([2.01437650e-03, 2.03441955e-01, 4.04869533e-01, 6.06297111e-01, 8.07724689e-01, 1.00915227e+00, 1.21057984e+00, 1.41200742e+00, 1.61343500e+00, 1.81486258e+00, 2.01629016e+00, 2.21771773e+00, 2.41914531e+00, 2.62057289e+00, 2.82200047e+00, 3.02342805e+00, 3.22485562e+00, 3.42628320e+00, 3.62771078e+00, 3.82913836e+00, 4.03056594e+00, 4.23199351e+00, 4.43342109e+00, 4.63484867e+00, 4.83627625e+00, 5.03770383e+00, 5.23913140e+00, 5.44055898e+00, 5.64198656e+00, 5.84341414e+00, 6.04484172e+00, 6.24626929e+00, 6.44769687e+00, 6.64912445e+00, 6.85055203e+00, 7.05197961e+00, 7.25340718e+00, 7.45483476e+00, 7.65626234e+00, 7.85768992e+00, 8.05911750e+00, 8.26054507e+00, 8.46197265e+00, 8.66340023e+00, 8.86482781e+00, 9.06625539e+00, 9.26768296e+00, 9.46911054e+00, 9.67053812e+00, 9.87196570e+00, 1.00733933e+01, 1.02748209e+01, 1.04762484e+01, 1.06776760e+01, 1.08791036e+01, 1.10805312e+01, 1.12819587e+01, 1.14833863e+01, 1.16848139e+01, 1.18862415e+01, 1.20876691e+01, 1.22890966e+01, 1.24905242e+01, 1.26919518e+01, 1.28933794e+01, 1.30948069e+01, 1.32962345e+01, 1.34976621e+01, 1.36990897e+01, 1.39005173e+01, 1.41019448e+01, 1.43033724e+01, 1.45048000e+01, 1.47062276e+01, 1.49076551e+01, 1.51090827e+01, 1.53105103e+01, 1.55119379e+01, 1.57133655e+01, 1.59147930e+01, 1.61162206e+01, 1.63176482e+01, 1.65190758e+01, 1.67205034e+01, 1.69219309e+01, 1.71233585e+01, 1.73247861e+01, 1.75262137e+01, 1.77276412e+01, 1.79290688e+01, 1.81304964e+01, 1.83319240e+01, 1.85333516e+01, 1.87347791e+01, 1.89362067e+01, 1.91376343e+01, 1.93390619e+01, 1.95404894e+01, 1.97419170e+01, 1.99433446e+01, 2.01447722e+01, 2.03461998e+01, 2.05476273e+01, 2.07490549e+01, 2.09504825e+01, 2.11519101e+01, 2.13533376e+01, 2.15547652e+01, 2.17561928e+01, 2.19576204e+01, 2.21590480e+01, 2.23604755e+01, 2.25619031e+01, 2.27633307e+01, 2.29647583e+01, 2.31661858e+01, 2.33676134e+01, 2.35690410e+01, 2.37704686e+01, 2.39718962e+01, 2.41733237e+01, 2.43747513e+01, 2.45761789e+01, 2.47776065e+01, 2.49790340e+01, 2.51804616e+01, 2.53818892e+01, 2.55833168e+01, 2.57847444e+01, 2.59861719e+01, 2.61875995e+01, 2.63890271e+01, 2.65904547e+01, 2.67918823e+01, 2.69933098e+01, 2.71947374e+01, 2.73961650e+01, 2.75975926e+01, 2.77990201e+01, 2.80004477e+01, 2.82018753e+01, 2.84033029e+01, 2.86047305e+01, 2.88061580e+01, 2.90075856e+01, 2.92090132e+01, 2.94104408e+01, 2.96118683e+01, 2.98132959e+01, 3.00147235e+01, 3.02161511e+01, 3.04175787e+01, 3.06190062e+01, 3.08204338e+01, 3.10218614e+01, 3.12232890e+01, 3.14247165e+01, 3.16261441e+01, 3.18275717e+01, 3.20289993e+01, 3.22304269e+01, 3.24318544e+01, 3.26332820e+01, 3.28347096e+01, 3.30361372e+01, 3.32375647e+01, 3.34389923e+01, 3.36404199e+01, 3.38418475e+01, 3.40432751e+01, 3.42447026e+01, 3.44461302e+01, 3.46475578e+01, 3.48489854e+01, 3.50504129e+01, 3.52518405e+01, 3.54532681e+01, 3.56546957e+01, 3.58561233e+01, 3.60575508e+01, 3.62589784e+01, 3.64604060e+01, 3.66618336e+01, 3.68632612e+01, 3.70646887e+01, 3.72661163e+01, 3.74675439e+01, 3.76689715e+01, 3.78703990e+01, 3.80718266e+01, 3.82732542e+01, 3.84746818e+01, 3.86761094e+01, 3.88775369e+01, 3.90789645e+01, 3.92803921e+01, 3.94818197e+01, 3.96832472e+01, 3.98846748e+01, 4.00861024e+01, 4.02875300e+01])
- (wavelength)float64counts583.995, 112.997, ..., 75.196, 4036.448σ = 24.166, 10.630, ..., 8.672, 63.533
Values:
array([5.83994750e+02, 1.12996750e+02, 8.79935000e+01, 1.96258210e+04, 3.40648233e+05, 8.22637958e+05, 1.18724871e+06, 1.54281212e+06, 2.01159275e+06, 2.79819798e+06, 4.17729281e+06, 6.19534679e+06, 8.06783862e+06, 8.67973603e+06, 8.61524345e+06, 8.33169573e+06, 7.80207555e+06, 7.27441804e+06, 6.79801052e+06, 6.49524456e+06, 6.77953889e+06, 6.53256041e+06, 6.16416695e+06, 5.82078621e+06, 5.45771476e+06, 5.02573205e+06, 4.60853168e+06, 4.21600198e+06, 3.84994400e+06, 3.51529047e+06, 3.20649829e+06, 2.92863274e+06, 2.67749343e+06, 2.44416365e+06, 2.23326159e+06, 2.04331749e+06, 1.87083061e+06, 1.71437872e+06, 1.57161893e+06, 1.44447600e+06, 1.33207641e+06, 1.22357308e+06, 1.13100293e+06, 1.04207938e+06, 9.62688388e+05, 8.87877156e+05, 8.23361576e+05, 7.65732862e+05, 7.09121801e+05, 6.59766386e+05, 6.13846448e+05, 5.72429791e+05, 5.31968672e+05, 4.95618554e+05, 4.65337135e+05, 4.33623983e+05, 4.05888277e+05, 3.78473305e+05, 3.54909728e+05, 3.33494837e+05, 3.12234804e+05, 2.94009137e+05, 2.76099528e+05, 2.59852750e+05, 2.43028645e+05, 2.17250609e+05, 1.22300140e+05, 3.42819600e+03, 1.87658250e+02, 8.90827500e+01, 8.78722500e+01, 7.52467500e+01, 6.39435000e+01, 8.18495000e+01, 6.61642500e+01, 7.38267500e+01, 8.79377500e+01, 7.81312500e+01, 7.89372500e+01, 8.91157500e+01, 7.29567500e+01, 7.18122500e+01, 6.91190000e+01, 8.00170000e+01, 7.70387500e+01, 7.10397500e+01, 8.68232500e+01, 6.90392500e+01, 8.00402500e+01, 7.48837500e+01, 1.04108750e+02, 7.30192500e+01, 7.48802500e+01, 5.72302500e+01, 7.97602500e+01, 8.58992500e+01, 8.30185000e+01, 6.71660000e+01, 7.01200000e+01, 7.89225000e+01, 7.09210000e+01, 7.19960000e+01, 7.59702500e+01, 7.60217500e+01, 7.71535000e+01, 7.37855000e+01, 6.91292500e+01, 7.70507500e+01, 6.99700000e+01, 6.09970000e+01, 7.08582500e+01, 8.10237500e+01, 6.72502500e+01, 7.47702500e+01, 7.89962500e+01, 7.89962500e+01, 7.80547500e+01, 8.07017500e+01, 6.42917500e+01, 6.49067500e+01, 7.00565000e+01, 7.69355000e+01, 8.10882500e+01, 6.30587500e+01, 8.68410000e+01, 6.20590000e+01, 8.30600000e+01, 6.70610000e+01, 7.46427500e+01, 7.60597500e+01, 6.43227500e+01, 7.67667500e+01, 8.19627500e+01, 8.20962500e+01, 7.20977500e+01, 5.29292500e+01, 6.49625000e+01, 7.58930000e+01, 7.69262500e+01, 6.50652500e+01, 7.30662500e+01, 6.40317500e+01, 9.26747500e+01, 8.12822500e+01, 6.22500000e+01, 7.07790000e+01, 7.31067500e+01, 7.01082500e+01, 7.35882500e+01, 7.71827500e+01, 6.70342500e+01, 8.27687500e+01, 8.22630000e+01, 5.91510000e+01, 8.48042500e+01, 7.39577500e+01, 6.19965000e+01, 6.59175000e+01, 5.32345000e+01, 7.17575000e+01, 7.29155000e+01, 7.11980000e+01, 7.47930000e+01, 6.03645000e+01, 7.17090000e+01, 8.51205000e+01, 8.90802500e+01, 6.40812500e+01, 6.90400000e+01, 7.49555000e+01, 8.56130000e+01, 5.75115000e+01, 7.46525000e+01, 7.80400000e+01, 7.21280000e+01, 5.97775000e+01, 7.79962500e+01, 7.80407500e+01, 7.67280000e+01, 7.33550000e+01, 7.69065000e+01, 6.49055000e+01, 6.01790000e+01, 7.28130000e+01, 7.00422500e+01, 9.08102500e+01, 8.08082500e+01, 7.19942500e+01, 7.53722500e+01, 7.11387500e+01, 7.19970000e+01, 5.62370000e+01, 7.97087500e+01, 7.78512500e+01, 8.29472500e+01, 6.60937500e+01, 8.01440000e+01, 7.59475000e+01, 7.51957500e+01, 4.03644775e+03])
Variances (σ²):
array([5.83994750e+02, 1.12996750e+02, 8.79935000e+01, 1.96258210e+04, 3.40648233e+05, 8.22637958e+05, 1.18724871e+06, 1.54281212e+06, 2.01159275e+06, 2.79819798e+06, 4.17729281e+06, 6.19534679e+06, 8.06783862e+06, 8.67973603e+06, 8.61524345e+06, 8.33169573e+06, 7.80207555e+06, 7.27441804e+06, 6.79801052e+06, 6.49524456e+06, 6.77953889e+06, 6.53256041e+06, 6.16416695e+06, 5.82078621e+06, 5.45771476e+06, 5.02573205e+06, 4.60853168e+06, 4.21600198e+06, 3.84994400e+06, 3.51529047e+06, 3.20649829e+06, 2.92863274e+06, 2.67749343e+06, 2.44416365e+06, 2.23326159e+06, 2.04331749e+06, 1.87083061e+06, 1.71437872e+06, 1.57161893e+06, 1.44447600e+06, 1.33207641e+06, 1.22357308e+06, 1.13100293e+06, 1.04207938e+06, 9.62688388e+05, 8.87877156e+05, 8.23361576e+05, 7.65732862e+05, 7.09121801e+05, 6.59766386e+05, 6.13846448e+05, 5.72429791e+05, 5.31968672e+05, 4.95618554e+05, 4.65337135e+05, 4.33623983e+05, 4.05888277e+05, 3.78473305e+05, 3.54909728e+05, 3.33494837e+05, 3.12234804e+05, 2.94009137e+05, 2.76099528e+05, 2.59852750e+05, 2.43028645e+05, 2.17250609e+05, 1.22300140e+05, 3.42819600e+03, 1.87658250e+02, 8.90827500e+01, 8.78722500e+01, 7.52467500e+01, 6.39435000e+01, 8.18495000e+01, 6.61642500e+01, 7.38267500e+01, 8.79377500e+01, 7.81312500e+01, 7.89372500e+01, 8.91157500e+01, 7.29567500e+01, 7.18122500e+01, 6.91190000e+01, 8.00170000e+01, 7.70387500e+01, 7.10397500e+01, 8.68232500e+01, 6.90392500e+01, 8.00402500e+01, 7.48837500e+01, 1.04108750e+02, 7.30192500e+01, 7.48802500e+01, 5.72302500e+01, 7.97602500e+01, 8.58992500e+01, 8.30185000e+01, 6.71660000e+01, 7.01200000e+01, 7.89225000e+01, 7.09210000e+01, 7.19960000e+01, 7.59702500e+01, 7.60217500e+01, 7.71535000e+01, 7.37855000e+01, 6.91292500e+01, 7.70507500e+01, 6.99700000e+01, 6.09970000e+01, 7.08582500e+01, 8.10237500e+01, 6.72502500e+01, 7.47702500e+01, 7.89962500e+01, 7.89962500e+01, 7.80547500e+01, 8.07017500e+01, 6.42917500e+01, 6.49067500e+01, 7.00565000e+01, 7.69355000e+01, 8.10882500e+01, 6.30587500e+01, 8.68410000e+01, 6.20590000e+01, 8.30600000e+01, 6.70610000e+01, 7.46427500e+01, 7.60597500e+01, 6.43227500e+01, 7.67667500e+01, 8.19627500e+01, 8.20962500e+01, 7.20977500e+01, 5.29292500e+01, 6.49625000e+01, 7.58930000e+01, 7.69262500e+01, 6.50652500e+01, 7.30662500e+01, 6.40317500e+01, 9.26747500e+01, 8.12822500e+01, 6.22500000e+01, 7.07790000e+01, 7.31067500e+01, 7.01082500e+01, 7.35882500e+01, 7.71827500e+01, 6.70342500e+01, 8.27687500e+01, 8.22630000e+01, 5.91510000e+01, 8.48042500e+01, 7.39577500e+01, 6.19965000e+01, 6.59175000e+01, 5.32345000e+01, 7.17575000e+01, 7.29155000e+01, 7.11980000e+01, 7.47930000e+01, 6.03645000e+01, 7.17090000e+01, 8.51205000e+01, 8.90802500e+01, 6.40812500e+01, 6.90400000e+01, 7.49555000e+01, 8.56130000e+01, 5.75115000e+01, 7.46525000e+01, 7.80400000e+01, 7.21280000e+01, 5.97775000e+01, 7.79962500e+01, 7.80407500e+01, 7.67280000e+01, 7.33550000e+01, 7.69065000e+01, 6.49055000e+01, 6.01790000e+01, 7.28130000e+01, 7.00422500e+01, 9.08102500e+01, 8.08082500e+01, 7.19942500e+01, 7.53722500e+01, 7.11387500e+01, 7.19970000e+01, 5.62370000e+01, 7.97087500e+01, 7.78512500e+01, 8.29472500e+01, 6.60937500e+01, 8.01440000e+01, 7.59475000e+01, 7.51957500e+01, 4.03644775e+03])
[11]:
sc.plot(data['monitors'], norm='log')
[11]:
[12]:
converted_monitors = {
f'monitor{i}': data['monitors'][f'monitor{i}'].transform_coords(
'wavelength', graph=wavelength_graph_monitor
)
for i in [1, 2, 3, 4, 5]
}
sc.plot(converted_monitors, norm='log')
[12]:
Step 4: Fixing metadata#
Exercise#
Consider the following (hypothetical) problems with the metadata stored in detector
:
The
sample_position
coord (detector.coords['sample_position']
) is wrong, shift the sample bydelta = sc.vector(value=np.array([0.01,0.01,0.04]), unit='m')
.Because of a glitch in the timing system the time-of-flight has an offset of \(2.3~\mu s\). Fix the corresponding coordinate.
Use the HTML view of
detector
to verify that you applied the corrections/calibrations there, rather than in a copy.
Solution#
[13]:
detector.coords['sample_position'] += sc.vector(value=[0.01, 0.01, 0.04], unit='m')
detector.coords['tof'] += 2.3 * sc.Unit(
'us'
) # note how we forgot to fix the monitor's TOF
detector
[13]:
- spectrum: 114688
- tof: 200
- position(spectrum)vector3m[ 0.778 0.13046651 29.85877813], [ 0.77506458 0.13046651 29.85877813], ..., [-5.69651663e-01 -2.28657089e-02 2.99532831e+01], [-5.72000000e-01 -2.28657089e-02 2.99532831e+01]
Values:
array([[ 7.78000000e-01, 1.30466508e-01, 2.98587781e+01], [ 7.75064579e-01, 1.30466508e-01, 2.98587781e+01], [ 7.72129159e-01, 1.30466508e-01, 2.98587781e+01], ..., [-5.67303327e-01, -2.28657089e-02, 2.99532831e+01], [-5.69651663e-01, -2.28657089e-02, 2.99532831e+01], [-5.72000000e-01, -2.28657089e-02, 2.99532831e+01]]) - sample_position()vector3m[1.000e-02 1.000e-02 2.534e+01]
Values:
array([1.000e-02, 1.000e-02, 2.534e+01]) - source_position()vector3m[0. 0. 0.]
Values:
array([0., 0., 0.]) - spectrum(spectrum)int32𝟙11, 12, ..., 114697, 114698
Values:
array([ 11, 12, 13, ..., 114696, 114697, 114698], dtype=int32) - tof(tof [bin-edge])float64µs7.3, 507.275, ..., 9.950e+04, 1.000e+05
Values:
array([7.3000000e+00, 5.0727500e+02, 1.0072500e+03, 1.5072250e+03, 2.0072000e+03, 2.5071750e+03, 3.0071500e+03, 3.5071250e+03, 4.0071000e+03, 4.5070750e+03, 5.0070500e+03, 5.5070250e+03, 6.0070000e+03, 6.5069750e+03, 7.0069500e+03, 7.5069250e+03, 8.0069000e+03, 8.5068750e+03, 9.0068500e+03, 9.5068250e+03, 1.0006800e+04, 1.0506775e+04, 1.1006750e+04, 1.1506725e+04, 1.2006700e+04, 1.2506675e+04, 1.3006650e+04, 1.3506625e+04, 1.4006600e+04, 1.4506575e+04, 1.5006550e+04, 1.5506525e+04, 1.6006500e+04, 1.6506475e+04, 1.7006450e+04, 1.7506425e+04, 1.8006400e+04, 1.8506375e+04, 1.9006350e+04, 1.9506325e+04, 2.0006300e+04, 2.0506275e+04, 2.1006250e+04, 2.1506225e+04, 2.2006200e+04, 2.2506175e+04, 2.3006150e+04, 2.3506125e+04, 2.4006100e+04, 2.4506075e+04, 2.5006050e+04, 2.5506025e+04, 2.6006000e+04, 2.6505975e+04, 2.7005950e+04, 2.7505925e+04, 2.8005900e+04, 2.8505875e+04, 2.9005850e+04, 2.9505825e+04, 3.0005800e+04, 3.0505775e+04, 3.1005750e+04, 3.1505725e+04, 3.2005700e+04, 3.2505675e+04, 3.3005650e+04, 3.3505625e+04, 3.4005600e+04, 3.4505575e+04, 3.5005550e+04, 3.5505525e+04, 3.6005500e+04, 3.6505475e+04, 3.7005450e+04, 3.7505425e+04, 3.8005400e+04, 3.8505375e+04, 3.9005350e+04, 3.9505325e+04, 4.0005300e+04, 4.0505275e+04, 4.1005250e+04, 4.1505225e+04, 4.2005200e+04, 4.2505175e+04, 4.3005150e+04, 4.3505125e+04, 4.4005100e+04, 4.4505075e+04, 4.5005050e+04, 4.5505025e+04, 4.6005000e+04, 4.6504975e+04, 4.7004950e+04, 4.7504925e+04, 4.8004900e+04, 4.8504875e+04, 4.9004850e+04, 4.9504825e+04, 5.0004800e+04, 5.0504775e+04, 5.1004750e+04, 5.1504725e+04, 5.2004700e+04, 5.2504675e+04, 5.3004650e+04, 5.3504625e+04, 5.4004600e+04, 5.4504575e+04, 5.5004550e+04, 5.5504525e+04, 5.6004500e+04, 5.6504475e+04, 5.7004450e+04, 5.7504425e+04, 5.8004400e+04, 5.8504375e+04, 5.9004350e+04, 5.9504325e+04, 6.0004300e+04, 6.0504275e+04, 6.1004250e+04, 6.1504225e+04, 6.2004200e+04, 6.2504175e+04, 6.3004150e+04, 6.3504125e+04, 6.4004100e+04, 6.4504075e+04, 6.5004050e+04, 6.5504025e+04, 6.6004000e+04, 6.6503975e+04, 6.7003950e+04, 6.7503925e+04, 6.8003900e+04, 6.8503875e+04, 6.9003850e+04, 6.9503825e+04, 7.0003800e+04, 7.0503775e+04, 7.1003750e+04, 7.1503725e+04, 7.2003700e+04, 7.2503675e+04, 7.3003650e+04, 7.3503625e+04, 7.4003600e+04, 7.4503575e+04, 7.5003550e+04, 7.5503525e+04, 7.6003500e+04, 7.6503475e+04, 7.7003450e+04, 7.7503425e+04, 7.8003400e+04, 7.8503375e+04, 7.9003350e+04, 7.9503325e+04, 8.0003300e+04, 8.0503275e+04, 8.1003250e+04, 8.1503225e+04, 8.2003200e+04, 8.2503175e+04, 8.3003150e+04, 8.3503125e+04, 8.4003100e+04, 8.4503075e+04, 8.5003050e+04, 8.5503025e+04, 8.6003000e+04, 8.6502975e+04, 8.7002950e+04, 8.7502925e+04, 8.8002900e+04, 8.8502875e+04, 8.9002850e+04, 8.9502825e+04, 9.0002800e+04, 9.0502775e+04, 9.1002750e+04, 9.1502725e+04, 9.2002700e+04, 9.2502675e+04, 9.3002650e+04, 9.3502625e+04, 9.4002600e+04, 9.4502575e+04, 9.5002550e+04, 9.5502525e+04, 9.6002500e+04, 9.6502475e+04, 9.7002450e+04, 9.7502425e+04, 9.8002400e+04, 9.8502375e+04, 9.9002350e+04, 9.9502325e+04, 1.0000230e+05])
- (spectrum, tof)float64counts0.0, 0.0, ..., 0.0, 0.0σ = 0.0, 0.0, ..., 0.0, 0.0
Values:
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
Variances (σ²):
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
Note how adding such offsets fails if we fail to specify a unit:
[14]:
detector.coords['tof'] += 2.3
---------------------------------------------------------------------------
UnitError Traceback (most recent call last)
Cell In[14], line 1
----> 1 detector.coords['tof'] += 2.3
UnitError: Cannot add µs and dimensionless.
This has several advantages: - We are protected from accidental errors. If someone changes the unit of data or metadata without our knowledge, e.g., from us
to ms
this mechanism protects us from silent errors corrupting the data. - It makes the code clearer and more readable, both for others as well as for our future selves.
The offset to the sample could also be done component-wise using the special fields
property of variables with “vector” dtype, e.g.,
[15]:
detector.coords['sample_position'].fields.z += 0.001 * sc.Unit('m')
Step 5: A closer look at the data#
In the very first task, we summed the data along the spectrum
dimension before plotting, yielding a pixel-integrated time-of-flight spectrum of the data. Here, we sum along the tof
dimension to inspect the contents of individual pixels:
[16]:
counts = detector.sum('tof')
Exercise#
Create a plot of
counts
and also try the instrument view.How many counts are there in total, in all spectra combined?
Plot a single spectrum of
detector
as a 1-D plot using the slicing syntaxarray[dim_name, integer_index]
to access the spectrum.
Solution#
[17]:
# slice is optional, making plot more readable in the documentation
counts['spectrum', 56000:62000].plot()
[17]:
[18]:
scn.instrument_view(counts, norm='log')
[18]:
[19]:
# detector.sum('spectrum') # would be another solution
detector.sum().value
[19]:
58142417.0
[20]:
detector['spectrum', 10000].plot()
[20]:
Volumetric detectors#
As seen in the instrument view the detectors consist of 4 layers of tubes, each containing 7 straws. Let us try to split up our data, so we can compare layers. There are other (and probably better) ways to do this, but here we try to define an integer variable containing a layer index:
[21]:
z = detector.coords['position'].fields.z
near = z.min()
far = z.max()
layer = ((z - near) * 400).astype('int32')
layer.unit = ''
layer.plot()
[21]:
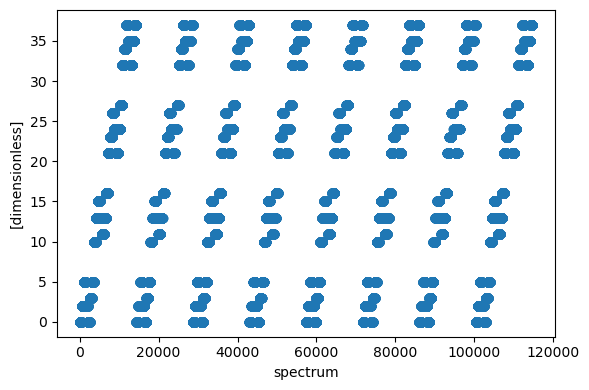
Exercise#
Change the magic parameter
400
in the cell above until pixels fall cleanly into layers, either 4 layers of tubes or 12 layers of straws.Store
layer
as a new coord indetector
.Use
detector.groupby(group='layer').sum('spectrum')
to group spectra into layers.Inspect and understand the HTML view of the result.
Plot the result. There are two options:
Use Plopp’s ``slicer` utility <https://scipp.github.io/plopp/plotting/slicer-plot.html>`__ to navigate the different layers using a slider (requires
%matplotlib widget
to enable interactive figures)Use
sc.plot
after collapsing dimensions,sc.collapse(grouped, keep='tof')
Bonus: When grouping by straw layers, there is a different number of straws in the center layer of each tube (3 instead of 2) due to the flower-pattern arrangement of straws. Define a helper data array with data set to 1 for each spectrum (using, e.g.,
norm = sc.DataArray(data=sc.ones_like(layer), coords={'layer':layer})
), group by layers and sum over spectrum as above, and use this result to normalize the layer-grouped data from above to spectrum count.
Solution#
[22]:
%matplotlib widget
# NOTE:
# - set magic factor to, e.g., 150 to group by straw layer
# - set magic factor to, e.g., 40 to group by tube layer
layer = ((z - near) * 150).astype(sc.DType.int32)
layer.unit = ''
detector.coords['layer'] = layer
grouped = detector.groupby(group='layer').sum('spectrum')
pp.slicer(grouped)
[22]:
[23]:
sc.plot(sc.collapse(grouped, keep='tof'))
[23]:
[24]:
norm = sc.DataArray(data=sc.ones_like(layer), coords={'layer': layer})
norm = norm.groupby(group='layer').sum('spectrum')
sc.plot(sc.collapse(grouped / norm, keep='tof'))
[24]: